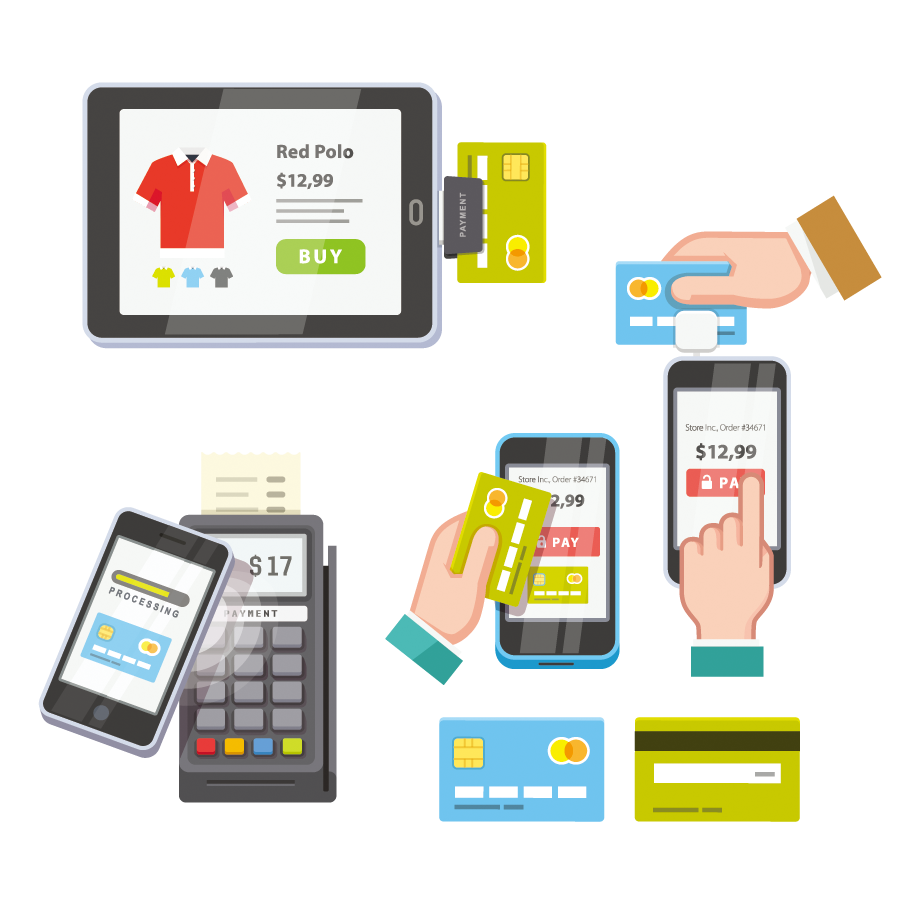
14
From Theory to Practice: Implementing UUIDv4 in Your Projects
This guide explores the theory and practical implementation of UUIDv4 in various projects. It covers the benefits of UUIDv4, how to generate it in different programming languages, and best practices for its use. Understanding UUIDv4 can help enhance data integrity, scalability, and compatibility in your projects.
In the world of software development, ensuring that every entity is uniquely identifiable is crucial. Universally Unique Identifiers (UUIDs) provide a robust solution for this need, and among the various versions, UUIDv4 stands out for its simplicity and effectiveness. This comprehensive guide will take you from the theory behind UUIDv4 to practical implementation in your projects, offering detailed insights to help you leverage this powerful tool.
Understanding UUIDv4
UUIDv4 is a 128-bit identifier used to uniquely identify information in computer systems. It is generated using random numbers, ensuring a high degree of uniqueness. The format of a UUIDv4 consists of 32 hexadecimal characters, divided into five groups separated by hyphens, following the pattern 8-4-4-4-12.
Benefits of Using UUIDv4
- Uniqueness: UUIDv4 provides a high level of uniqueness, making it ideal for applications that require unique keys, such as databases and distributed systems.
- Simplicity: Generating a UUIDv4 is straightforward and does not require complex algorithms or dependencies. Most programming languages and frameworks offer built-in functions to generate UUIDv4s.
- Scalability: UUIDv4 can be generated independently on different systems without the need for a central authority, making it suitable for distributed environments.
- Compatibility: UUIDv4 is widely supported across various platforms and technologies, ensuring compatibility and interoperability between different systems.
Implementing UUIDv4 in Your Projects
- Generating UUIDv4 in Different Programming Languages:
- Python:
import uuid unique_id = uuid.uuid4() print(unique_id)
- JavaScript:
const { v4: uuidv4 } = require('uuid'); const uniqueId = uuidv4(); console.log(uniqueId);
- Java:
import java.util.UUID; UUID uniqueId = UUID.randomUUID(); System.out.println(uniqueId);
- PHP:
$uniqueId = uniqid(); echo $uniqueId;
Using UUIDv4 in Databases:
- MySQL:
CREATE TABLE users ( id CHAR(36) PRIMARY KEY, name VARCHAR(255) NOT NULL ); INSERT INTO users (id, name) VALUES (UUID(), 'John Doe');
- PostgreSQL:
CREATE TABLE users ( id UUID PRIMARY KEY, name VARCHAR(255) NOT NULL ); INSERT INTO users (id, name) VALUES (uuid_generate_v4(), 'John Doe');
- UUIDv4 in Distributed Systems:
- In distributed systems, UUIDv4 can be used to uniquely identify nodes, transactions, and messages. This helps maintain consistency and traceability in complex environments.
- UUIDv4 in API Development:
- UUIDv4 is often used in API development to uniquely identify resources, such as users, orders, and products. This ensures that each resource can be accessed and managed independently.
Best Practices for Using UUIDv4
- Avoid Sequential UUIDs: While UUIDv4 is designed to be random, some implementations may generate sequential UUIDs. Ensure that your UUID generation method produces truly random identifiers.
- Use UUIDv4 for Unique Keys: UUIDv4 is ideal for use as primary keys in databases, especially in distributed environments where uniqueness across different systems is required.
- Validate UUIDs: Implement validation checks to ensure that UUIDs conform to the expected format. This helps prevent errors and ensures data integrity.
- Monitor Performance: While UUIDv4 is efficient, generating and storing large numbers of UUIDs can impact performance. Monitor your system's performance and optimize as needed.
Conclusion
Implementing UUIDv4 in your projects can enhance data integrity, scalability, and compatibility. By understanding the theory behind UUIDv4 and following best practices for its implementation, you can leverage this powerful tool to create robust and reliable systems. Whether you're working with databases, distributed systems, or APIs, UUIDv4 provides a simple and effective solution for generating unique identifiers.
Contact
Missing something?
Feel free to request missing tools or give some feedback using our contact form.
Contact Us